A software engineering program provides higher education in the field of software engineering. Software engineering is a discipline involved in developing and maintaining software systems. These systems are used in various industries, such as telecommunications, healthcare, finance, and many more. A software engineering program typically includes coursework in computer science, software development, and project management.
A software engineering program can be beneficial for those who want to work in the software industry. The program provides students with the knowledge and skills needed to develop, test, and maintain software systems. Graduates of a software engineering program are prepared to work in a variety of roles in the software industry, such as software developer, software engineer, and software architect.
The history of software engineering programs can be traced back to the early days of computer science. In the 1960s, the first computer science programs were developed at universities in the United States and the United Kingdom. These programs included coursework in software development, and they provided students with the skills needed to develop software systems for a variety of applications.
Software Engineering Program
A software engineering program provides students with the knowledge and skills needed to develop, test, and maintain software systems. These programs are typically offered at colleges and universities, and they can be completed in four years or less. Software engineering programs cover a wide range of topics, including computer science, software development, and project management. Graduates of software engineering programs are prepared to work in a variety of roles in the software industry, such as software developer, software engineer, and software architect.
- Computer science: The study of the theory and practice of computation.
- Software development: The process of designing, developing, and testing software systems.
- Project management: The process of planning, executing, and completing software projects.
- Data structures: The organization and storage of data in a computer system.
- Algorithms: The step-by-step instructions that a computer follows to solve a problem.
- Software testing: The process of evaluating the correctness, completeness, and quality of software.
- Software maintenance: The process of keeping software systems up-to-date and running smoothly.
- Software engineering ethics: The study of the ethical implications of software development and use.
- Software engineering best practices: The recommended practices for developing and maintaining software systems.
These are just a few of the key aspects of a software engineering program. By studying these topics, students will gain the knowledge and skills needed to develop high-quality software systems that meet the needs of users.
Computer science
Computer science is the foundation of software engineering. It provides the theoretical and practical knowledge that is essential for developing software systems. Without a strong foundation in computer science, it would be impossible to design, develop, and maintain complex software systems.
- Algorithms and data structures: Algorithms are the step-by-step instructions that a computer follows to solve a problem. Data structures are the way that data is organized and stored in a computer. Both algorithms and data structures are essential for developing efficient and effective software systems.
- Software design: Software design is the process of creating a blueprint for a software system. This blueprint includes the system's architecture, components, and interfaces. Software design is essential for ensuring that a software system is well-structured, maintainable, and extensible.
- Software testing: Software testing is the process of evaluating the correctness, completeness, and quality of software. Software testing is essential for ensuring that a software system meets its requirements and is free of defects.
- Software engineering ethics: Software engineering ethics is the study of the ethical implications of software development and use. Software engineering ethics is essential for ensuring that software systems are developed and used in a responsible and ethical manner.
These are just a few of the ways that computer science is connected to software engineering. By studying computer science, software engineers gain the knowledge and skills needed to develop high-quality software systems that meet the needs of users.
Software development
Software development is a critical component of a software engineering program. It is the process of designing, developing, and testing software systems. Software development is a complex and challenging process that requires a deep understanding of computer science principles and software engineering best practices.
The software development process typically begins with requirements gathering. This involves understanding the needs of the users and stakeholders who will be using the software system. Once the requirements have been gathered, the software development team will create a design for the system. The design will specify the system's architecture, components, and interfaces.
Once the design is complete, the software development team will begin developing the system. This involves writing code, testing the code, and integrating the code into the system. The development process is iterative, meaning that the team will repeat these steps until the system is complete.
Once the system is complete, the software development team will test the system to ensure that it meets the requirements. The testing process will involve running tests on the system to identify any defects. The team will then fix any defects that are found.
Once the system has been tested and is free of defects, the software development team will deploy the system to the users. The deployment process involves installing the system on the users' computers and providing training on how to use the system.
Software development is a complex and challenging process, but it is also a critical process for developing high-quality software systems. By understanding the software development process, software engineers can develop systems that meet the needs of users and stakeholders.
Project management
Project management is a critical component of software engineering programs. It provides students with the knowledge and skills needed to plan, execute, and complete software projects successfully. Project management is essential for ensuring that software projects are completed on time, within budget, and to the required quality standards.
- Planning: The planning phase of a software project involves defining the project scope, objectives, and schedule. It also involves identifying the resources that will be needed to complete the project.
- Execution: The execution phase of a software project involves carrying out the tasks that were defined in the planning phase. This includes developing the software, testing the software, and deploying the software.
- Completion: The completion phase of a software project involves closing out the project and delivering the final product to the customer. This includes documenting the project, evaluating the project, and archiving the project artifacts.
Project management is a complex and challenging process, but it is essential for the successful completion of software projects. By understanding the project management process, software engineers can increase their chances of completing projects on time, within budget, and to the required quality standards.
Data structures
Data structures are a fundamental part of computer science and software engineering. They provide a way to organize and store data in a computer system so that it can be accessed and processed efficiently. Without data structures, it would be very difficult to develop complex software systems that can handle large amounts of data.
- Arrays: Arrays are a simple but powerful data structure that can store a collection of data items of the same type. Arrays are often used to store data that is related in some way, such as a list of names or a list of numbers.
- Linked lists: Linked lists are a more complex data structure that can store a collection of data items that are not necessarily related. Linked lists are often used to store data that is constantly changing, such as a list of tasks or a list of customers.
- Stacks: Stacks are a data structure that follows the last-in, first-out (LIFO) principle. This means that the last item that is added to the stack is the first item that is removed. Stacks are often used to store data that needs to be processed in a specific order, such as a list of function calls.
- Queues: Queues are a data structure that follows the first-in, first-out (FIFO) principle. This means that the first item that is added to the queue is the first item that is removed. Queues are often used to store data that needs to be processed in a first-come, first-served order, such as a list of customers waiting for service.
These are just a few of the many different data structures that are available. Each data structure has its own advantages and disadvantages, and the choice of which data structure to use depends on the specific needs of the application.
Algorithms
Algorithms are an essential part of software engineering. They are the step-by-step instructions that a computer follows to solve a problem. Without algorithms, computers would not be able to perform even the simplest tasks.
Algorithms are used in all aspects of software engineering, from designing the overall architecture of a software system to implementing individual features. For example, algorithms are used to:
- Sort data
- Search for data
- Optimize performance
- Control the flow of a program
The study of algorithms is a core part of a software engineering program. Students learn how to design and implement efficient algorithms for a variety of problems. This knowledge is essential for developing high-quality software systems that meet the needs of users.
For example, in a software engineering program, students might learn about different sorting algorithms, such as bubble sort, insertion sort, and merge sort. They would learn how to analyze the efficiency of these algorithms and choose the best algorithm for a particular problem.
Algorithms are a fundamental part of software engineering. They are the building blocks of software systems. By understanding algorithms, software engineers can develop more efficient, reliable, and maintainable software systems.
Software testing
Software testing is an essential part of the software engineering process. It helps to ensure that software is correct, complete, and of high quality. Software testing can be performed manually or using automated tools.
Manual testing involves executing the software and checking the results against the expected results. Automated testing uses tools to execute the software and check the results. Automated testing is more efficient and can be used to test more complex software systems.
Software testing is important because it helps to identify and fix defects in software. Defects can cause software to crash, produce incorrect results, or behave in unexpected ways. By identifying and fixing defects, software testing helps to ensure that software is reliable and meets the needs of users.
In a software engineering program, students learn about software testing principles and techniques. They also gain experience in performing software testing. This knowledge and experience is essential for software engineers who want to develop high-quality software.
For example, in a software engineering program, students might learn about different types of software testing, such as unit testing, integration testing, and system testing. They would also learn how to use software testing tools and how to write effective test cases.
Software testing is an important part of the software engineering process. It helps to ensure that software is correct, complete, and of high quality. Software testing is a valuable skill for software engineers who want to develop high-quality software.
Software maintenance
Software maintenance is a critical part of the software engineering process. It involves keeping software systems up-to-date and running smoothly. Software maintenance is important because it helps to ensure that software systems are reliable, secure, and meet the needs of users.
- Correcting defects: Software maintenance involves correcting defects in software. Defects can be caused by errors in the software code, changes in the operating environment, or new user requirements. Software maintenance engineers use a variety of techniques to identify and correct defects, including testing, debugging, and code analysis.
- Updating software: Software maintenance also involves updating software to new versions. New versions of software may include new features, bug fixes, or security patches. Software maintenance engineers must carefully test and deploy new software versions to ensure that they do not cause any problems.
- Adapting software: Software maintenance also involves adapting software to new environments or requirements. For example, a software maintenance engineer may need to adapt software to a new operating system or to support a new hardware device. Software maintenance engineers must have a deep understanding of the software system and its environment in order to successfully adapt the software.
- Improving performance: Software maintenance can also involve improving the performance of software. Software maintenance engineers may use a variety of techniques to improve performance, such as optimizing the software code, tuning the database, or adding hardware resources.
Software maintenance is a complex and challenging process, but it is essential for keeping software systems up-to-date and running smoothly. Software maintenance engineers play a critical role in ensuring that software systems are reliable, secure, and meet the needs of users.
Software engineering ethics
Software engineering ethics is the study of the ethical implications of software development and use. It is a relatively new field, but it is becoming increasingly important as software becomes more and more pervasive in our lives.
Software engineering ethics raises a number of important questions, such as:
- What are the ethical obligations of software engineers?
- How can we ensure that software is used for good and not for evil?
- How can we protect the privacy of users?
- How can we prevent software from being used to discriminate against people?
These are just a few of the many ethical issues that software engineers must consider. By studying software engineering ethics, software engineers can learn how to develop and use software in a responsible and ethical manner.
Software engineering ethics is an important component of a software engineering program. It helps students to understand the ethical implications of their work and to make ethical decisions about the software they develop.
For example, a software engineering ethics course might cover topics such as:
- The history of software engineering ethics
- The ethical principles that should guide software engineers
- The ethical issues that arise in the development and use of software
- The role of software engineers in society
By studying these topics, students can learn how to identify and resolve ethical issues that arise in the development and use of software.
Software engineering ethics is a complex and challenging field, but it is essential for ensuring that software is developed and used in a responsible and ethical manner.
Software engineering best practices
Software engineering best practices are a set of guidelines and recommendations that software engineers can follow to improve the quality and reliability of their software. These best practices cover a wide range of topics, including software design, development, testing, and maintenance. By following software engineering best practices, software engineers can help to ensure that their software is well-structured, maintainable, and bug-free. Software engineering best practices are an essential part of a software engineering program. They provide students with the knowledge and skills they need to develop high-quality software. By learning and applying software engineering best practices, students can improve their chances of success in the software industry.
There are many benefits to following software engineering best practices. For example, following best practices can help to:
- Improve the quality of software
- Reduce the number of bugs in software
- Make software easier to maintain
- Improve the performance of software
- Reduce the cost of developing software
In addition, following software engineering best practices can help to improve the safety and security of software. By following best practices, software engineers can help to reduce the risk of software failures and security breaches.
Software engineering best practices are a valuable resource for software engineers. By following best practices, software engineers can improve the quality, reliability, and security of their software.
Here are some examples of software engineering best practices:
- Use a version control system to track changes to your code.
- Write unit tests for your code.
- Use a bug tracking system to track and fix bugs.
- Document your code.
- Follow a coding style guide.
By following these best practices, software engineers can help to ensure that their software is of high quality and is easy to maintain.
FAQs on Software Engineering Programs
This section provides answers to frequently asked questions about software engineering programs, addressing common concerns and misconceptions.
Question 1: What is a software engineering program?A software engineering program is a higher education program that equips students with the knowledge and skills necessary to design, develop, test, and maintain software systems. These programs typically cover various aspects of software engineering, including computer science, software development, and project management.
Question 2: What are the benefits of completing a software engineering program?Graduates of software engineering programs are highly sought after in the tech industry, as they possess the expertise and Fhigkeiten to develop innovative and reliable software solutions. They have a competitive advantage in securing roles as software developers, software engineers, and software architects.
Question 3: What are the career prospects for software engineers?Software engineers enjoy a wide range of career opportunities in diverse industries, including technology, finance, healthcare, and manufacturing. They can work in various settings, such as research and development, product development, and technical support.
Question 4: What are the admission requirements for software engineering programs?Admission requirements vary among institutions, but most programs typically require a strong foundation in mathematics, science, and computer programming. Applicants may also need to submit standardized test scores and personal statements.
Question 5: What is the duration of a software engineering program?The duration of a software engineering program typically ranges from three to five years, depending on the institution and the specific program structure. Some programs offer accelerated options, allowing students to complete their studies in a shorter time frame.
Question 6: What are the costs associated with a software engineering program?The costs of a software engineering program vary widely depending on factors such as the institution, program duration, and location. Students should research and compare the costs of different programs to make informed decisions.
Overall, software engineering programs provide a comprehensive education that prepares students for successful careers in the dynamic field of software development. By equipping graduates with the necessary knowledge, skills, and industry connections, these programs play a vital role in shaping the future of software engineering.
Transition to the next article section...
Software Engineering Program Tips
Enrolling in a software engineering program can provide you with the foundation and skills essential for a successful career in software development. Here are some valuable tips to consider:
Tip 1: Choose a Reputable Program:
Research and compare different software engineering programs to identify those with a strong reputation, experienced faculty, and industry connections. Consider factors such as program accreditation, curriculum relevance, and graduate employment rates.
Tip 2: Focus on Core Concepts:
Pay particular attention to mastering core computer science and software engineering principles. These include data structures, algorithms, software design patterns, and development methodologies. A solid understanding of these concepts will serve as the cornerstone for your future success.
Tip 3: Enhance Practical Skills:
Complement theoretical knowledge with practical experience through hands-on projects, internships, and open-source contributions. Building a portfolio of applied work will demonstrate your proficiency and make you a more attractive candidate for employers.
Tip 4: Collaborate with Peers:
Software engineering often involves teamwork. Actively engage in group projects and discussions to develop your collaboration, communication, and problem-solving skills. These abilities are highly valued in the industry.
Tip 5: Stay Updated with Trends:
The field of software engineering is constantly evolving. Make a conscious effort to stay abreast of emerging technologies, best practices, and industry trends. This will ensure that your knowledge and skills remain relevant throughout your career.
Tip 6: Seek Mentorship:
Identify experienced software engineers or professors who can provide guidance and support. Mentors can offer valuable insights, career advice, and help you navigate the challenges of the profession.
Tip 7: Participate in Extracurricular Activities:
Involvement in software engineering clubs, competitions, or hackathons can supplement your academic learning. These activities provide opportunities to network, showcase your skills, and gain exposure to real-world software development challenges.
Tip 8: Develop a Strong Work Ethic:
Software engineering requires hard work, dedication, and perseverance. Develop a strong work ethic and be prepared to invest time and effort into your studies and projects. This will pay dividends in the long run.
By following these tips, you can maximize your experience in a software engineering program and prepare yourself for a rewarding career in this dynamic and ever-growing field.
Transition to the article's conclusion...
Software Engineering Program
Software engineering programs equip individuals with the knowledge, skills, and mindset to excel in the dynamic field of software development. Through rigorous academic coursework, hands-on experience, and industry engagement, these programs empower graduates to design, develop, and maintain innovative software solutions that shape our world.
As technology continues to advance at an unprecedented pace, the demand for skilled software engineers will only intensify. By investing in a software engineering program, you are not only pursuing a rewarding career but also becoming an active participant in shaping the future of technology. Embrace the challenges, stay curious, and let your passion for software engineering drive you towards excellence.
Youtube Video:
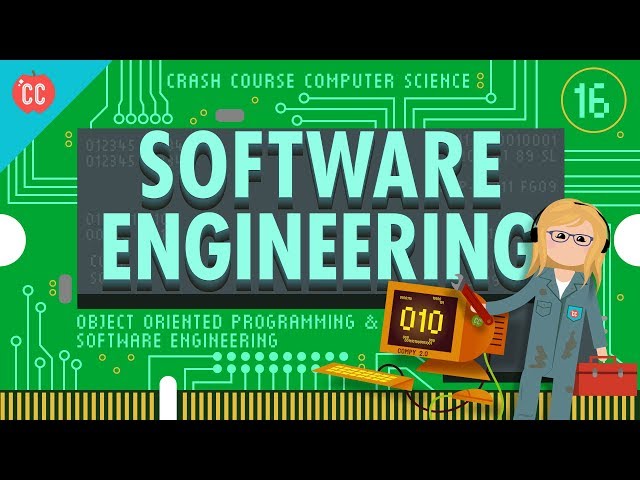